This tutorial will show you the making of the ReadRatio WordPress plugin.
If you need to integrate an external JavaScript file into WordPress via a plugin, keep reading.
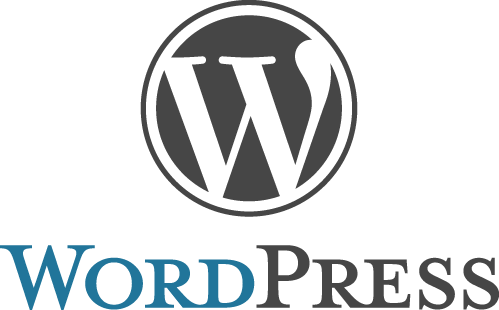
The plugin will:
- Add a script tag on all pages
- Add a custom setting in the WordPress admin dashboard
For the background, ReadRatio is my latest project, a web analytics tool designed for bloggers.
If you're new to WordPress plugin development, you should start by reading the Writing a Plugin guide on the WordPress Codex.
The directory structure
Since this is a basic plugin, we will fit everything into a single PHP file. Here is how I structured my project:
.
├── Makefile
├── README.md
└── readratio
├── readme.txt
└── readratio.php
1 directory, 4 files
Hooking the admin dashboard
Our first goal is to let the user input a custom setting in the admin dashboard.
Adding settings is easy, we just need to use add_action with the admin_init
tag.
The WordPress Plugin Handbook explains it very well so take a look at Registering a Setting section for more details.
<?php
function readratio_settings_api_init() {
add_settings_section(
'readratio_setting_section',
'ReadRatio Settings',
'readratio_setting_section_callback_function',
'general'
);
add_settings_field(
'readratio_website_id',
'ReadRatio Website ID',
'readratio_setting_callback_function',
'general',
'readratio_setting_section'
);
register_setting( 'general', 'readratio_website_id' );
}
add_action( 'admin_init', 'readratio_settings_api_init' );
function readratio_setting_section_callback_function() {
?>
<p>You can get your <strong>website id</strong> in the <strong>Tracking Code</strong> section on <a href="https://readratio.com">ReadRatio</a>.</p>
<?php
}
function readratio_setting_callback_function() {
$setting = esc_attr( get_option( 'readratio_website_id' ) );
echo "<input type='text' name='readratio_website_id' value='$setting' />";
}
See the result:
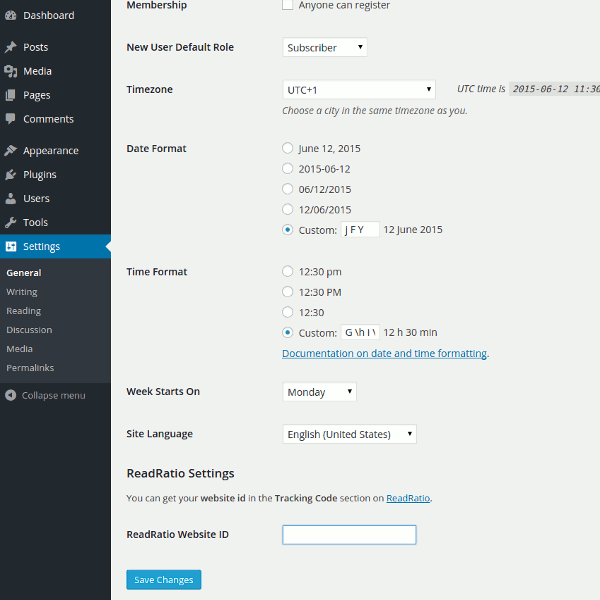
Including the script
The second goal is to insert an HTML snippet into every page.
There is two way to achieve this:
- wp_enqueue_script, if you need to include a single script, without any setting.
- add_action with the
wp_footer
tag, it will insert the output of the callback function into the footer.
I will show you the add_action
method since it let you access previously saved settings.
<?php
function readratio_scripts() {
$setting = esc_attr( get_option( 'readratio_website_id' ) );
if ($setting) {
?>
<script type="text/javascript">
var _readratio = _readratio || [];
_readratio.push(['_setID', <?php echo json_encode( $setting ); ?>]);
(function() {
var b = document.createElement('script'); b.type = 'text/javascript'; b.async = true;
b.src = 'https://d1zhan3dzk8vm3.cloudfront.net/readratio.min.js';
var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(b, s);
})();
</script>
<?php
}
}
add_action( 'wp_footer', 'readratio_scripts' );
If you want to load a script locally, you will need to use plugins_url to help you find your script url.
Installing the widget
To install the widget, you have to upload the zipped directory in your admin dashboard.
Let's zip the plugin:
$ zip -r "readratio.zip" readratio
Source Code
The ReadRatio WordPress plugin that inspired this article is open source and hosted on GitHub.
Useful Links
- Writing a Plugin, the official documentation on the WordPress codex.
- readme.txt generator, if you publish your plugin, you need a readme.txt file.
Bonus: Start a WordPress sandbox with Docker
If you have Docker installed on your server, using the "Out-of-the-box" WordPress docker image by Tutum is a quick way to start a clean WordPress instance.
$ docker run -d -p 8001:80 tutum/wordpress
This will listen on port 8001.
Bonus: The Makefile
Plugins are usually distributed as a zip file, this basic Makefile will produce the gip file and use the git tag to name the file i.e. readratio-v0.0.1.zip
.
V=$(shell git describe --tags)
all:
-rm readratio-$(V).zip
zip -r "readratio-$(V).zip" readratio
Your feedback
Please share your feedback or any tip you might have!
Tip with Bitcoin
Tip me with Bitcoin and vote for this post!
Leave a comment